Analytics¶
We provide a complete C# API for all the analytics events required by our analytics guys.
Setup¶
Our backend solution to capture user data is Amplitude. In order to talk to their SDK, we must provide application api keys.
You should already have a development and a production keys. If this is not the case, please contact your producer.
Your CoreApplication component should have an AnalyticsManager
component, where you can specify these keys.
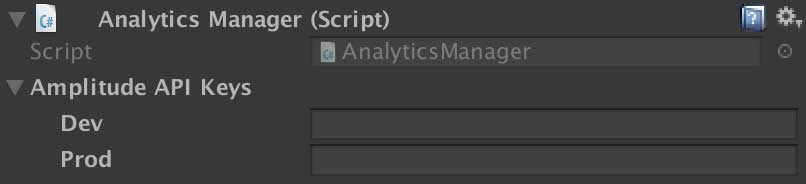
Basic Usage¶
Sending an event is quite simple:
- Retrieve the
AnalyticsManager
from theCoreApplication
. - Log the event, by giving it’s name and additional key/value properties.
AnalyticsManager analyticsManager = CoreApplication.Instance.AnalyticsManager;
analyticsManager.LogEvent(string eventType, IDictionary<string, object> eventProperties);
Cross Platform¶
Amplitude provides a Unity SDK, including the iOS and Android native SDKs. To complete this, we developped the AmplitudeHttp
service which can talk directly to their REST API. This is completly transparent when you use the AnalyticsManager
.
Custom Asmodee events¶
In order to properly analyse your game in production, our analytics guys need to probe your code for all kind of user actions, user properties and game events.
Header¶
Most of HEADER information is already set, but you should provide:
analyticsManager.SetVersionBuildNumber(string value) // version_build_number
Event properties¶
You should provide the following data:
analyticsManager.SetBackendPlatform(string value) // backend_platform
analyticsManager.SetBackendUserId(string value) // backend_user_id
analyticsManager.SetUAPlatform(string value) // ua_platform
analyticsManager.SetUAUserId(string value) // ua_user_id
analyticsManager.SetUAChannel(string value) // ua_channel
analyticsManager.SetPushPlatform(string value) // push_platform
analyticsManager.SetPushUserId(string value) // push_user_id
analyticsManager.SetFirstParty(string value) // first_party
analyticsManager.SetUserIdFirstParty(string value) // user_id_first_party
analyticsManager.SetABTestGroup(string value) // ab_test_group
analyticsManager.SetKarma(int? value) // karma
analyticsManager.SetEloRating(int? value) // elo_rating
You can also add your own specific user properties via:
analyticsManager.SetUserProperties(IDictionary<string, object> userProperties)
Simple events¶
Some events are fire and forget:
AnalyticsEvents.LogFindMeEvent() // FIND_ME
AnalyticsEvents.LogAppBootEvent(APP_BOOT.launch_method launchMethod) // APP_BOOT
AnalyticsEvents.LogHeartbeatEvent() // HEARTBEAT
AnalyticsEvents.LogAdDisplayEvent(string adType, bool isSkippable, bool isSkipped, int timeSec, string adTrigger, string contextId) // AD_DISPLAY
AnalyticsEvents.LogContentUnlockEvent(string contentId, string contentType, string unlockReason) // CONTENT_UNLOCK
AnalyticsEvents.LogAchievementUnlockEvent(string achievementId) // ACHIEVEMENT_UNLOCK
AnalyticsEvents.LogFriendManagementEvent(string action, string friendUserId, int friendCount) // FRIEND_MANAGEMENT
AnalyticsEvents.LogDebugEvent(bool isVisible, string messageType, string errorCode, string messageContent) // DEBUG
AnalyticsEvents.LogTutorialStepEvent(int tutorialVersion, string stepId, float stepSequenceNumber, TUTORIAL_STEP.step_status stepStatus, int timeOnStep, bool isTutoComplete) // TUTORIAL_STEP
AnalyticsEvents.LogSettingChangeEvent(string setting, string valueOld, string valueNew) // SETTING_CHANGE
AnalyticsEvents.LogCustomerSupportDisplayedEvent(string openPath, string errorMessage)) // CUSTOMER_SUPPORT_DISPLAYED
AnalyticsEvents.LogGetUseDropEvent(string perspective, bool direction, string contextId, string itemFrom, string itemTo, string mapId, string matchSessionId, string shopSessionId, string itemUnit, bool isRealCurrency, string itemInstanceId, string itemId, string itemType, float? usesRemaining, float quantity, string txId) // GET_USE_DROP
Complexe events¶
Other events require to keep a context, save some data, compute time delta. They usally come with a start*** and stop*** tags.
Cross Promo
AnalyticsEvents.LogCrossPromoOpenedEvent(CROSSPROMO_OPENED.crosspromo_type? crossPromoType) // CROSSPROMO_OPENED
AnalyticsEvents.LogCrossPromoRedirectedEvent(ShowcaseProduct product, CROSSPROMO_REDIRECTED.product_type productType) // CROSSPROMO_REDIRECTED
Content download
AnalyticsEvents.LogContentDlStartEvent(string dlContentId) // CONTENT_DL_START
AnalyticsEvents.LogContentDlStopEvent(bool dlIsComplete, CONTENT_DL_STOP.dl_end_reason dlEndReason) // CONTENT_DL_STOP
Match
AnalyticsEvents.LogMatchStartEvent(string matchSessionId, string lobbySessionId, string mode, string mapId, string[] activatedDlc, int playerCountHuman, int playerCountAi, int? playerPlayOrder, string launchMethod, int? playerClockSec, string difficulty, bool isOnline, bool isTutorial, bool? isAsynchronous, bool? isPrivate, bool? isRanked, bool? isObservable, MATCH_START.obs_access? obsAccess, bool? obsShowHiddenInfo, bool? isObserver) // MATCH_START
AnalyticsEvents.LogMatchStopEvent(int playerCountHuman, int playerCountAi, string endReason, MATCH_STOP.player_result? playerResult, int? turnCount) // MATCH_STOP
Lobby
AnalyticsEvents.LogLobbyStartEvent(int? onlinePlayerCountConnected, int? onlinePlayerCountLobbyOrTable, int? onlinePlayerCountTable, int? onlinePlayerCountMatch, int? onlineOpenTableCount, int? onlineOngoingMatchCount) // LOBBY_START
AnalyticsEvents.LogLobbyStopEvent(int? onlinePlayerCountConnected, int? onlinePlayerCountLobbyOrTable, int? onlinePlayerCountTable, int? onlinePlayerCountMatch, int? onlineOpenTableCount, int? onlineOngoingMatchCount, string endReason) // LOBBY_STOP
Table
AnalyticsEvents.LogTableStartEvent(string matchSessionId, string lobbySessionId, string launchMethod, int playerCountSlots, int playerCountHuman, int playerCountAi, int? playerClockSec, bool? isAsynchronous, bool? isPrivate, bool? isRanked, bool? isObservable, TABLE_START.obs_access? obsAccess, bool? obsShowHiddenInfo) // TABLE_START
AnalyticsEvents.LogTableStopEvent(string endReason, int playerCountSlots, int playerCountHuman, int playerCountAi) // TABLE_STOP
Screen Display
AnalyticsEvents.LogScreenDisplayEvent(string name, string screenPreviousNavAction) // SCREEN_DISPLAY
Shop
AnalyticsEvents.LogShopStartEvent(string entryPoint, string itemId) // SHOP_START
AnalyticsEvents.LogShopItemViewEvent(string itemId, float itemPrice, string itemCurrency) // SHOP_ITEM_VIEW
AnalyticsEvents.LogShopItemPurchaseEvent(string itemId, float itemPrice, string itemCurrency, int itemQuantity, string transactionBackendId, string transactionFirstPartyId)
AnalyticsEvents.LogShopStopEvent() // SHOP_STOP
Asmodee.net Account
AnalyticsEvents.LogConnectAsmodeeNetStartEvent(string connectPath) // CONNECT_ASMODEENET_START
AnalyticsEvents.LogConnectAsmodeeNetStopEvent(bool isSigninComplete, string lastError, bool resetPassword) // CONNECT_ASMODEENET_STOP
AnalyticsEvents.LogCreateAsmodeeNetAccountEvent(bool isEmailOptIn) // CREATE_ASMODEENET_ACCOUNT